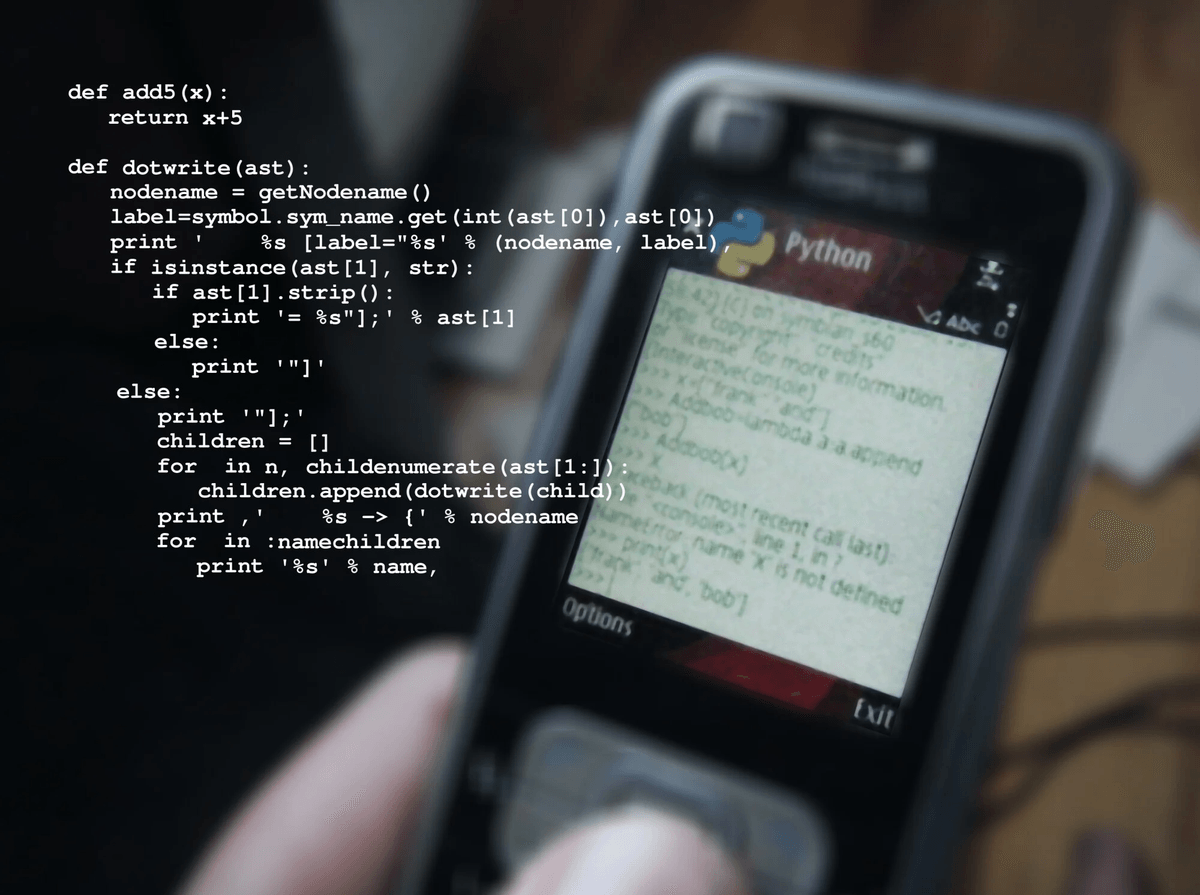
Python's abs module is a built-in module that provides a function to calculate the absolute value of a number. The absolute value of a number is its distance from zero, and it is always positive. In this article, we will explore the power of Python's abs module and how it can be used in various scenarios.
Understanding the Abs Function
The abs function is a simple yet powerful function that takes a single argument, which is the number for which you want to calculate the absolute value. The function returns the absolute value of the number, which is always positive. For example, if you pass the number -5 to the abs function, it will return 5.
Using the Abs Function
The abs function can be used in a variety of scenarios. For example, you can use it to calculate the distance between two points on a number line. You can also use it to normalize a number, which means to convert it to a value between 0 and 1.
Here is an example of how you can use the abs function to calculate the distance between two points on a number line:
```
x1 = 3
x2 = -4
distance = abs(x2 - x1)
print(distance) # Output: 7
```
In this example, the abs modules are used to calculate the distance between the points 3 and -4 on a number line. The output is 7, which is the distance between the two points.
Another example of how you can use the abs function is to normalize a number. Here is an example:
```
x = -0.5
normalized_x = abs(x) / max(abs(x), 1)
print(normalized_x) # Output: 0.5
```
In this example, the abs function is used to calculate the absolute value of the number -0.5. The result is then divided by the maximum of the absolute value and 1, which normalizes the number to a value between 0 and 1.
The Power of the Abs Module
The abs module is not just limited to the abs function. It also provides a way to calculate the absolute value of complex numbers. Complex numbers are numbers that have both real and imaginary parts. The abs module provides a function called cmath, which is used to calculate the absolute value of complex numbers.
Here is an example of how you can use the cmath function to calculate the absolute value of a complex number:
```
import cmath
z = complex(-3, 4)
abs_z = cmath.abs(z)
print(abs_z) # Output: 5.0
```
In this example, the cmath function is used to calculate the absolute value of the complex number -3 + 4i. The output is 5.0, which is the absolute value of the complex number.
Conclusion
In conclusion, Python's abs module is a powerful tool that provides a way to calculate the absolute value of numbers and complex numbers. The abs function is a simple yet powerful function that can be used in a variety of scenarios, from calculating distances between points on a number line to normalizing numbers. The cmath function is used to calculate the absolute value of complex numbers, which is an important concept in mathematics and science. With the abs module, you can perform a wide range of mathematical operations and calculations with ease.